C++ URI Parser: Your Comprehensive Guide for Effective Software Development
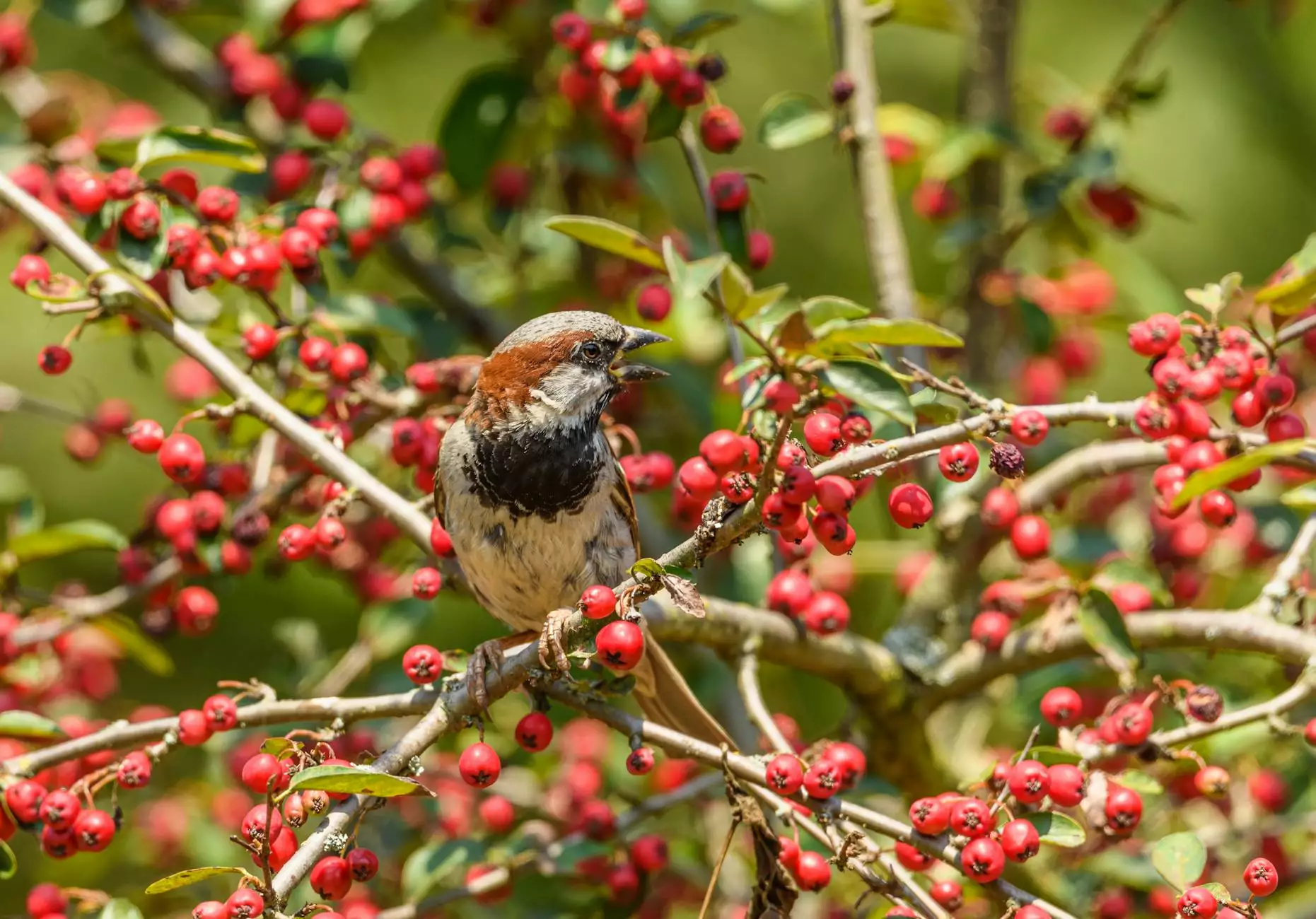
The world of software development is vast and full of possibilities, especially when it comes to web technologies. One of the fundamental components that aspiring developers should familiarize themselves with is the C++ URI Parser. This article delves deep into the intricacies of URI parsing in C++, shedding light on its significance in modern web design and software development.
Understanding URIs and Their Importance
A Uniform Resource Identifier (URI) is a string of characters that uniquely identifies a particular resource on the internet. It plays a crucial role in web development by allowing various applications to locate resources effectively. Know more about why URIs are essential:
- Resource Identification: URIs help pinpoint specific resources, facilitating efficient information retrieval.
- Standardization: They provide a standardized way for different applications to reference resources on the web.
- Interoperability: URIs enable different software systems to work together, which is crucial for web services and applications.
What is a C++ URI Parser?
A C++ URI Parser is a specialized tool designed to interpret and manipulate URIs within C++ programs. It enables developers to extract components from a URI, validate URIs, and even reconstruct them when necessary. The parser can handle various URI formats and is essential in building reliable web applications.
Key Components of a URI
Understanding the structure of a URI is vital when developing a parser. Typically, a URI consists of the following components:
- Scheme: Indicates the protocol used (e.g., http, https, ftp).
- Host: Represents the domain name or IP address of the resource.
- Port: Specifies the port number of the server (optional).
- Path: Refers to the specific location of the resource on the server.
- Query: A string of additional parameters used for retrieving specific information.
- Fragment: Points to a specific part of a resource (optional).
Benefits of Using C++ for URI Parsing
Choosing C++ for developing a URI parser comes with a plethora of advantages, which include:
- Performance: C++ is known for its speed and efficiency, making it suitable for high-performance applications.
- Control: It provides granular control over memory management, which is beneficial for optimizing resource usage.
- Portability: C++ code can be easily ported across different platforms, helping maintain a consistent experience for users.
- Rich Libraries: The availability of numerous libraries enhances the capabilities of C++ in URI handling.
How to Implement a C++ URI Parser
Implementing a C++ URI Parser requires a good understanding of both C++ syntax and the logic behind URI structures. Below, we outline a basic implementation approach:
Step 1: Define the URI Structure
The first step in creating a C++ URI parser is to define a structure that will hold the URI components. Here’s a simple example:
struct URI { std::string scheme; std::string host; int port; std::string path; std::string query; std::string fragment; };Step 2: Parsing the URI
The next step is to write a function that can take a string input (the full URI) and parse it into the defined structure. A basic version of this function could look like this:
URI parseURI(const std::string& uri) { URI result; // Sample parsing logic based on regex or string manipulation // Populate the result structure with parsed components return result; }Step 3: Validating the URI
Once parsed, it's crucial to validate the URI components to ensure they adhere to URIs' rules:
bool isValidURI(const URI& uri) { // Implement validation logic for each component return true; // or false based on validation }Real-World Applications of C++ URI Parsers
C++ URI Parsers are utilized in various real-world scenarios, including:
- Web Browsers: URI parsers are integral to web browsers for resolving and displaying web resources.
- APIs: They help in parsing request URLs and managing query parameters in RESTful APIs.
- Network Applications: In network programming, parsing URIs is essential for establishing connections and data transfers.
- Data Serialization: In applications dealing with data interchange formats, ensuring that URIs are correctly formatted is critical.
Challenges in C++ URI Parsing
While implementing a C++ URI parser, developers may face several challenges:
- Complexity of URIs: Some URIs include a vast array of edge cases that need to be handled delicately.
- Encoding Issues: Developers must account for percent-encoding and other encodings used in URIs.
- Thread Safety: Ensuring that the parser is thread-safe if used in a multi-threaded environment.
Enhancing Your C++ URI Parsing Skills
To master C++ URI parsing, consider the following approaches:
- Study URI Standards: Familiarize yourself with specifications such as RFC 3986, which defines the syntax of URIs.
- Practice: Build various projects requiring URI parsing to solidify your understanding.
- Contribute to Open Source: Engage with existing open-source C++ URI parser projects to learn and improve your skills.
Conclusion
In today’s highly digital world, understanding how to manage and parse URIs using C++ is an invaluable skill for any developer. The C++ URI Parser not only enhances application functionality but also streamlines web interactions. By implementing robust parsing strategies and understanding the underlying principles, developers can significantly improve their software development projects. Embrace the power of C++ and the potential of URI parsing to build efficient, reliable web applications that stand out in the competitive landscape of web design and software development.
For those interested in advancing their skills further, platforms like semalt.tools offer resources in Web Design and Software Development, ensuring you have access to the best tools and knowledge to excel in your programming journey.